Android control color RGB LED using HC-05 Bluetooth with Arduino (Part I)
Part II of this tutorial is here!
Updated Sketch for ESP32 boards https://stonez56.blogspot.com/2021/07/android-control-color-rgb-led-with.html
This time, I am going to show you how to use Android phone to connect to HC-05 Bluetooth module and Arduino.
Hardware needed:
- Arduino mini pro * 1
- HC-05 Bluetooth module * 1
- RGB LED * 2
- Jump wires * 10
HC-05 Bluetooth Module wiring as follow:
- TX connect to Arduino Pin 11(RX)
- RX connect to Arduino Pin 12(TX)
- Remember to connect TX on the HC-05 to RX on the Arduino and RX on the HC-05 to TX on the Arduino, so Arduino can receive data correctly.
- VCC connect to 5V power
- GND connect to Ground
RGB LED wiring as follow:
Warning: You must connect Arduino mini pro, so your PWM Pin can control LED’s color! For example, Arduino mini pro only has 6 PWM pins and they are 3, 5, 6, 9, 10, and 11, other pins do not have PWM.Refer to Wikipedia for PWM
- R connect to Arduino Pin 3
- Common Anode connect to 5V
Note: if you bought common anode LED, then this pin must connect to VCC 5V. If you bought common cathode LED, then this pin must connect to GND - G connect to Arduino Pin 5
- B connect to Arduino Pin 6
Arduino wiring as follow:
- GND connect to Ground
- VCC connect to 5V
If you connect all the parts together, it will look like this:
The completed Arduino code is listed here:
#include <Softwareserial.h> #include <Wire.h>//Include libraries: SoftwareSerial & Wire SoftwareSerial BT(11,12); //Define PIN11 & PIN12 as RX and TX pins //RGB LED Pins int PIN_RED = 3; int PIN_GREEN = 5; int PIN_BLUE = 6; //RED LED at Pin 13 int RED_LED = 13; String RGB = ""; //store RGB code from BT String RGB_Previous = "255.255.255)"; //preserve previous RGB color for LED switch on/off, default White String ON = "ON"; //Check if ON command is received String OFF = "OFF"; //Check if OFF command is received boolean RGB_Completed = false; void setup() { Serial.begin(9600); //Arduino serial port baud rate:9600 BT.begin(9600);//My HC-05 module default baud rate is 9600 RGB.reserve(30); pinMode(RED_LED, OUTPUT); //Set pin13 as output for LED, // this LED is on Arduino mini pro, not the RGB LED } void loop() { // put your main code here, to run repeatedly: //Read each character from Serial Port(Bluetooth) while(BT.available()){ char ReadChar = (char)BT.read(); // Right parentheses ) indicates complet of the string if(ReadChar == ')'){ RGB_Completed = true; }else{ RGB += ReadChar; } } //When a command code is received completely with ')' ending character if(RGB_Completed){ //Print out debug info at Serial output window Serial.print("RGB:"); Serial.print(RGB); Serial.print(" PreRGB:"); Serial.println(RGB_Previous); if(RGB==ON){ digitalWrite(13,HIGH); RGB = RGB_Previous; //We only receive 'ON', so get previous RGB color back to turn LED on Light_RGB_LED(); }else if(RGB==OFF){ digitalWrite(13,LOW); RGB = "0.0.0)"; //Send OFF string to turn light off Light_RGB_LED(); }else{ //Turn the color according the color code from Bluetooth Serial Port Light_RGB_LED(); RGB_Previous = RGB; } //Reset RGB String RGB = ""; RGB_Completed = false; } //end if of check if RGB completed } // end of loop void Light_RGB_LED(){ int SP1 = RGB.indexOf('.'); int SP2 = RGB.indexOf('.', SP1+1); int SP3 = RGB.indexOf('.', SP2+1); String R = RGB.substring(0, SP1); String G = RGB.substring(SP1+1, SP2); String B = RGB.substring(SP2+1, SP3); //Print out debug info at Serial output window Serial.print("R="); Serial.println( constrain(R.toInt(),0,255)); Serial.print("G="); Serial.println(constrain(G.toInt(),0,255)); Serial.print("B="); Serial.println( constrain(B.toInt(),0,255)); //Light up the LED with color code //**2014-09-21 //Because these RGB LED are common anode (Common positive) //So we need to take 255 to minus R,G,B value to get correct RGB color code analogWrite(PIN_RED, (255-R.toInt())); analogWrite(PIN_GREEN, (255-G.toInt())); analogWrite(PIN_BLUE, (255-B.toInt())); }
You need to go to Google Play Store and download "BT LED Controller"; you need to use it with this tutorial. You may download APP here
This is what Android BT LED Controller App can do:
1. Connect to Bluetooth2. Wireless control LED on/off
3. Wireless control LED to change the color
The screen of Android APP is below and you can use it easily:
- Click the ”BT-List” button (The red dot next to it means BT not connected)
- Next select the Bluetooth you want to connect from the list, after it connected successfully, the APP will jump back to the main screen
- On the main screen says BT connected (The green dot indicates BT connected)
- Click LED on / LED off button, you can turn LED on/off
- Click any color block on the App, the RGB color code will be sent to HC-05 and HC-05 will send the RGB color code to Arduino. RGB code is composed 3 numbers of 1~255 with “)” as the ending character, such as “255.20.10)”. Arduino receives a character at a time from the serial port and it combines all characters together. When a “)” is received, the RGB code is completed.
- Arduino then decompose RGB code string into 3 sets of numbers for R, G, B, and then send the code to RGB LED to display the color indicated.
- As shown below (RGB code 255.51.255 will make RGB LED to show magenta)
- Click Disconnect to cut the connection between Android and Arduino.
You also learn how to use this APP by watching
the video below:
Android 藍芽連線控制彩色 RGB LED (Part I)
本次教學將如何用 Android 程式以藍芽方式連線到HC-05藍芽模組及Arduino.
硬體所需材料:
- Arduino mini pro * 1
- HC-05 Bluetooth module * 1
- RGB LED * 2
- Jump wires * 10
HC-05 Module 接法:
- TX接到 Arduino Pin 11 (RX)
- RX接到 Arduino Pin 12 (TX)
就是發射端與接收端要反接到Arduino, 這樣 Arduino 才收的到訊號 - VCC 接 5V 電源
- GND 接地
RGB LED 接法:
注意: 一定要接 Arduino mini pro 有 PWM 的腳位才能控制LED的顏色!
以 Arduino mini pro PWM 腳位只有 : 3, 5, 6, 9, 10, and 11,其它沒有 PWM.
- R 接 Arduino Pin 3
- Common Anode 接 5V
(我買的是共陽的 LED, 所以這支腳要接 5V,若你買的是共陰的 Cathode, 則要接 GND) - G 接 Arduino Pin 5
- B 接 Arduino Pin 6
Arduino 接法:
- GND 接地
- VCC 接 5V
Arduino, HC-05 module, & RGB LED 電路圖如下:
我把這些材料通通接好如下:
請由 Google Play Store 下載 BT_LED_Controller V1.0 與這個程式搭配這個教學使用.
由此下載 APP
Android BT_LED_Controller V1.0 有下列功能:
- 藍芽連線
- 無線遙控 LED 開/關
- 無線遙控 LED 改變它的顏色
Android App 畫面如下,使用方法很簡單:
- 點選上方 "BT-LIST" 鈕 (右方的小紅點表示藍芽尚未連線)
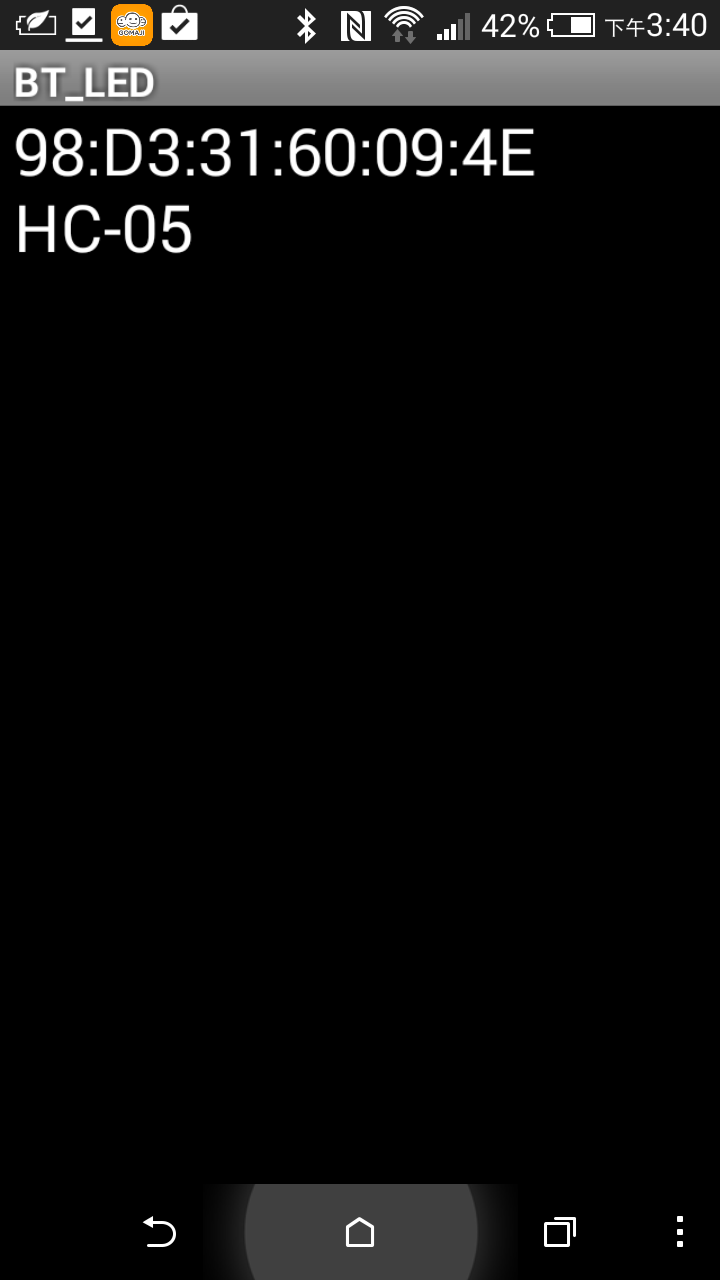
- 主畫面上顯示 BT Connected (小綠點表示已藍芽連線成功)
- 按下 LED Turn on / LED Turn Off 可以打開/關閉 LED
- 點選 App 上的任一顏色方塊,App 會把那個方塊的 RGB code 送到 HC-05,再由 HC-05傳給 Arduino, RGB code 由3組 1~255的數字組成, 再加上右括號尚作是結束符號, 如 255.20.10), 當 Arduino 由 serial port 一個字元一個字元接收, 一直收到 ) 時,才當作是一個完整的 RGB code。
- Arduino 把 RGB code 字串解析後,再送給 RGB LED 顯示指定的顏色
如下圖 (RGB code: 255.51.255 會傳到到 Arduino 顯示出紫紅色) - 點選 Disconnect 則關閉 Android 和 Arduino 的連線
- 完成測試
請參考底下的影片顯示操作的方法: